Anyone who has worked with a code base backed by the Spring Framework has probably encountered RestTemplate
at some point: a simple HTTP client which allows type-based consumption of HTTP endpoints:
restTemplate.getForObject("https://some.api/employee/1", Employee.class);
Circa 2017, Spring 5 introduced WebFlux. Reactive Java was just picking up steam and the Spring Framework accommodated for this demand by offering it as a modern alternative to Web MVC. Part of this new reactive stack was WebClient which offered an alternative to RestTemplate
: functional (akin to Stream) and with reactive support to accommodate a more async approach for consuming web APIs.
Though being reactive, WebClient
still supported blocking non-async operations. This rambling is not about the reactive hype cycle reactive vs. imperative but rather how due to a combination of circumstances the common misconception was born that RestTemplate
was already deprecated.
Changed Javadoc
Root cause seems to be that with Spring 5.0.9, the RestTemplate
javadoc got updated to note that WebClient
is the modern alternative to RestTemplate
and RestTemplate
will eventually be deprecated:
WebClient offers a modern alternative to the RestTemplate [...] RestTemplate will be deprecated in a future version.
Almost 2 years and a few minor versions later (with Spring 5.2.4) the note was changed and no longer mentioned any future deprecation. Instead, it marked RestTemplate
as future-complete and offered WebClient as an alternative:
As of 5.0 this class is in maintenance mode, with only minor requests for changes and bugs to be accepted going forward. Please, consider using the WebClient which has a more modern API and supports sync, async, and streaming scenarios.
At this point, the damage was already done. Due to a long period of time with the inconsistent and confusingly worded javadoc, there is lots of dust on the internet treating RestTemplate as being deprecated; various blogs, StackOverflow questions and online discussions explicitly mention this.
@Deprecated
. The Spring Framework is quite rigorous with semantic versioning and backwards compatibility and real deprecations are almost always announced in a timely fashion with a clear migration schedule.The non-reactive codebase with reactive sauce
Personally, I have also encountered this in the "wild" where developers were anxious to keep using RestTemplate
and instead felt they had to use WebClient
because "everyone says RestTemplate
is deprecated!". This often lead to a non-reactive codebase being dipped into WebFlux sauce: blocking code everywhere but due to the spring-boot-starter-webflux
dependency, reactive elements seeped into the codebase.
Sadly, this sometimes escalated into a slippery slope where developers would keep adding more reactive concepts until they were drowning in obtuse stack traces and hard to understand concepts without any noticeable benefit. A recent reddit thread inquiring about the usage of Spring WebFlux has an amusing anecdote:
Had an epic to remove it.
- Wide-Syllabub3430
I'm not saying that the reactive model was a mistake, but with the improvements of virtual threads it probably becomes even a less attractive option than before.
RestTemplate back in action: RestClient
Having said all of that, RestTemplate
is now really back on the menu. Preferably not by using it directly but rather through the newly introduced RestClient which ships with Spring Boot 3.2 (Spring Framework 6.1). Instead of all of the overloaded methods RestTemplate has, the API is a lot more fluent but under the hood uses all the concepts and infrastructure of RestTemplate
:
Employee someEmployee = restClient.get()
.uri("https://some.api/employee/1")
.retrieve()
.body(Employee.class);
This way, you can use a relatively simple HTTP client with a modern API without having to include any webflux
dependency or call .block()
at the end of every operation.
The note in the javadoc of RestTemplate has also been updated to reflect this:
As of 6.1,RestClient
offers a more modern API for synchronous HTTP access. For asynchronous and streaming scenarios, consider the reactiveWebClient
Conclusion
The years of depreciation warnings and the common misconception will probably not be undone by the introduction of the new RestClient
. Amusingly, even as recent as in the issue discussing the introduction of this new client, someone hops along inquiring about RestTemplate
being deprecated but is quickly corrected nonetheless:
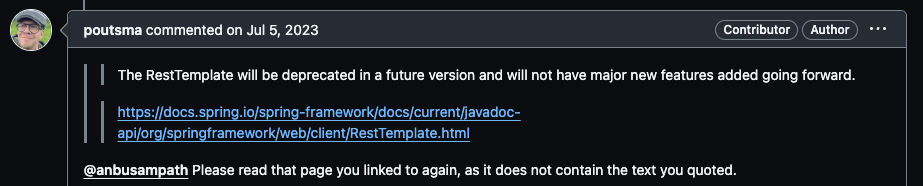
Given the blast radius of the Spring Framework, the impact of a notice in their javadoc is completely different from the javadoc that is typically written in a codebase not used all over the world. Either way, RestTemplate
was never truly deprecated. But you probably want to use RestClient
if you're on a recent Spring version. Or WebClient
if you really need reactive asynchronicity.
Want more content like this?
Hi! I sporadically write about tech & business. If you want an email when something new comes out, feel free to subscribe. It's free and I hate spam as much as you do.